一、百度换肤
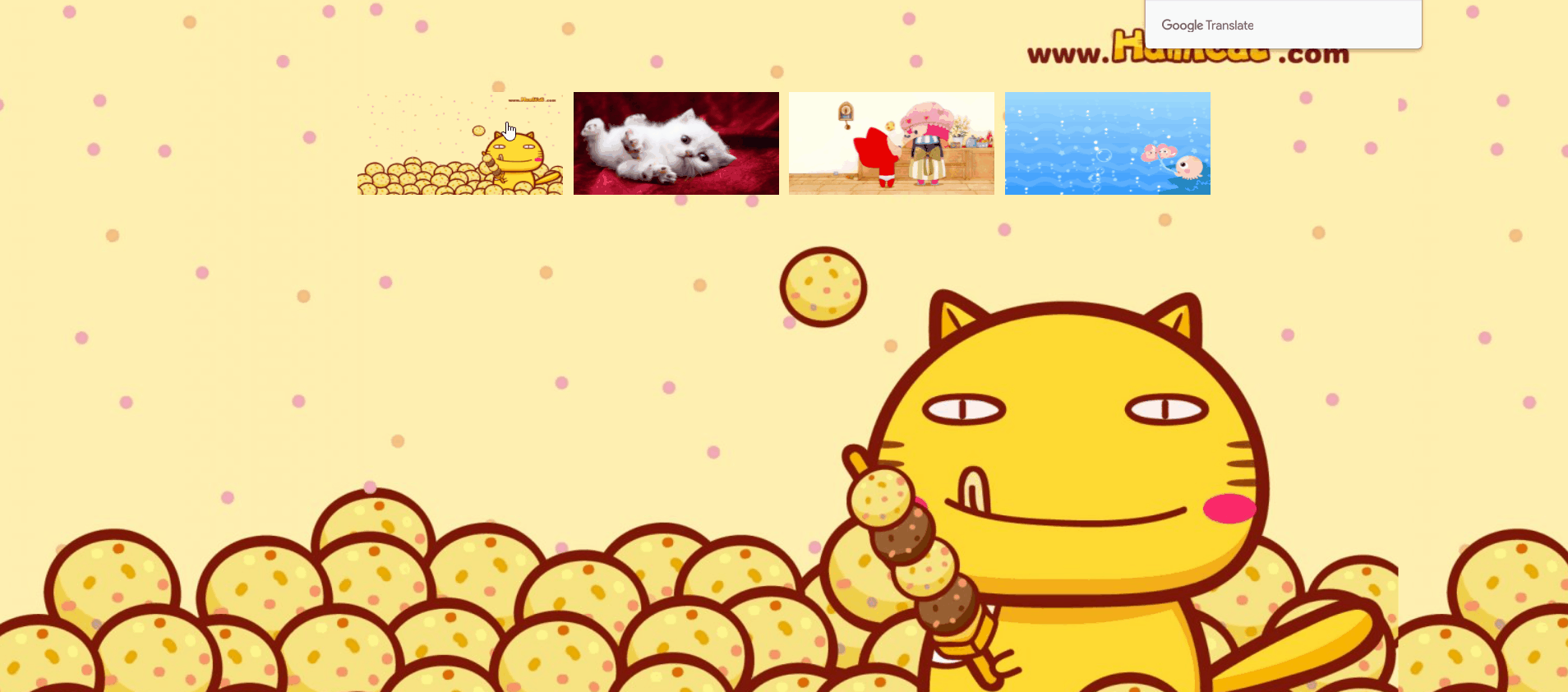
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
*{
margin: 0px;
padding: 0px;
list-style: none;
}
ul{
width: 830px;
height: 100px;
margin: 100px auto;
}
li{
float: left;
margin-right: 10px;
width:200px;
height: 100px;
cursor: pointer;
}
li:nth-of-type(4){
margin-right: 0px;
}
li img{
width: 200px;
height: 100px;
}
body{
background: url('../images/01.jpg');
}
</style>
</head>
<body>
<ul>
<li><img src="../images/01.jpg" alt=""></li>
<li><img src="../images/02.jpg" alt=""></li>
<li><img src="../images/03.jpg" alt=""></li>
<li><img src="../images/04.jpg" alt=""></li>
</ul>
<script src="../js/jquery.min.js"></script>
<script>
$('li').click(function(){
var picture = $(this).children().attr("src");
$('body').css("background","url("+picture+")");
});
</script>
</body>
</html>
二、表格
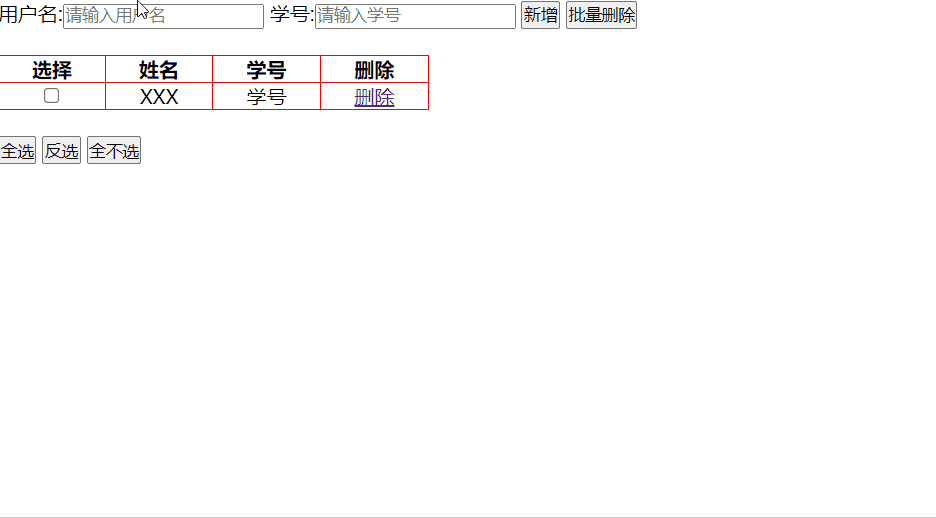
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0px;
padding: 0px;
list-style: none;
}
table {
border-collapse: collapse;
width: 345px;
height: 30px;
}
th,
td {
border: 1px solid red;
text-align: center;
}
</style>
</head>
<body>
用户名:<input type="text" placeholder="请输入用户名">
学号:<input type="text" placeholder="请输入学号">
<button>新增</button>
<button>批量删除</button>
<br><br>
<table>
<tr>
<th>选择</th>
<th>姓名</th>
<th>学号</th>
<th>删除</th>
</tr>
<tr>
<td><input type="checkbox" class="check"></td>
<td>XXX</td>
<td>学号</td>
<td><a href="#">删除</a></td>
</tr>
</table>
<br>
<button>全选</button>
<button>反选</button>
<button>全不选</button>
<script src="../js/jquery.min.js"></script>
<script>
function del(delList) {
$(delList).parent().parent().remove();
}
$('a').click(function () {
del(this);
});
$('button').eq(0).click(function () {
// 新增
var txt = $('input').eq(0).val();
var number = $('input').eq(1).val();
if(txt == '' || number == ''){
alert('内容为空,请重新输入');
}
else{
var newList = $('<tr></tr>');
$(newList).html(`<td><input type="checkbox" class='check'></td>
<td>${txt}</td>
<td>${number}</td>
<td><a href="#">删除</a></td>`);
$(newList).children('td').eq(3).children().click(function(){
del(this);
});
$('tr').eq(0).after(newList);
$('input').eq(0).val('');
$('input').eq(1).val('');
}
});
$('button').eq(1).click(function () {
//批量删除
$(':checked').parent().parent().remove();//:checked 选取所有选中的复选框或单选按钮。
});
$('button').eq(2).click(function () {
// 全选
$('.check').prop('checked',true);
//使用jQuery 的attr() 方法设置选择框 checked 失效核心原因是:property 和 attribute 实时同步的原因 。
/*
其解决办法:
方法1:使用 $(选择器).prop(“checked ”,true),进行设置 。
方法2:使用JS 获得元素,设置checked属性 为 true(选中) 或 false (不选中)
*/
// prop()方法设置或返回被选元素的属性和值。
});
$('button').eq(3).click(function () {
// 反选
// for(var i = 0;i<$('.check').length;i++){
// $('.check').eq(i).prop('checked',!$('.check').eq(i).prop('checked'));
// }
var select = $(':checked');
//not():方法返回不符合一定条件的元素 :checkbox选取类型为 checkbox 的 <input> 元素
var notSelect = $(':checkbox').not(':checked');
select.prop('checked',false);
notSelect.prop('checked',true);
});
$('button').eq(4).click(function () {
// 全不选
$('.check').prop('checked',false);
});
</script>
</body>
</html>
三、抽奖
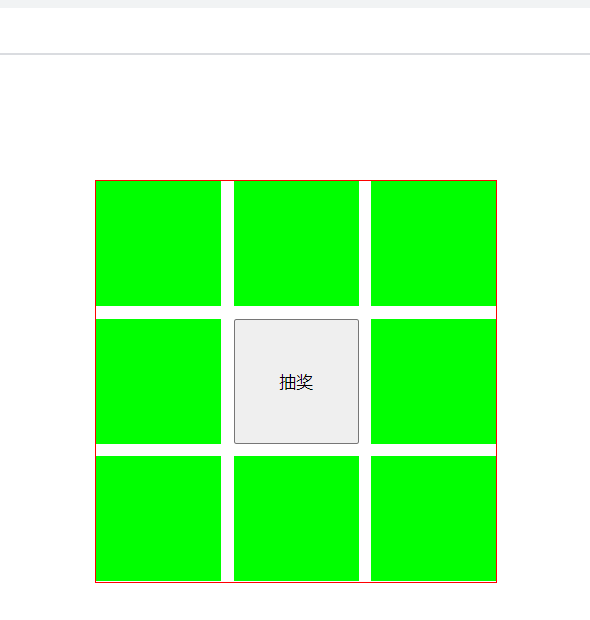
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0px;
padding: 0px;
list-style: none;
}
div {
width: 320px;
height: 320px;
margin: 100px auto;
border: 1px solid red;
position: relative;
}
ul {
width: 320px;
height: 320px;
}
li {
width: 100px;
height: 100px;
background: lime;
position: absolute;
}
li:nth-of-type(1) {
top: 0px;
left: 0px;
}
li:nth-of-type(2) {
top: 0px;
left: 110px;
}
li:nth-of-type(3) {
top: 0px;
left: 220px;
}
li:nth-of-type(4) {
top: 110px;
left: 220px;
}
li:nth-of-type(5) {
top: 220px;
left: 220px;
}
li:nth-of-type(6) {
top: 220px;
left: 110px;
}
li:nth-of-type(7) {
top:220px;
left: 0px;
}
li:nth-of-type(8) {
top:110px;
left: 0px;
}
button{
position: absolute;
top:110px;
left: 110px;
width:100px;
height: 100px;
outline:none;
}
.current{
opacity: 0.3;
}
</style>
</head>
<body>
<div>
<ul class="box">
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
<button>抽奖</button>
</div>
<script src="../js/jquery.min.js"></script>
<script>
var num = -1;
var sum = 0;
var timer;
function move(){
var round = parseInt((Math.random()*8)+1);
clearInterval(timer);
timer = setInterval(function(){
num++;
if(num>8){
num = 0;
sum++;
}
$('.box').children('li').eq(num).addClass('current');
$('.box').children('li').eq(num).siblings().removeClass('current');
if(sum >= 3){
if(num+1>=round){
clearInterval(timer);
sum = 0;
num = -1;
setTimeout(function(){
alert('第'+($('.current').index()+1)+'个位置');
$('.current').removeClass('current');
},200)
}
}
},50)
}
$('button').click(function(){
move();
});
</script>
</body>
</html>
四、轮播图
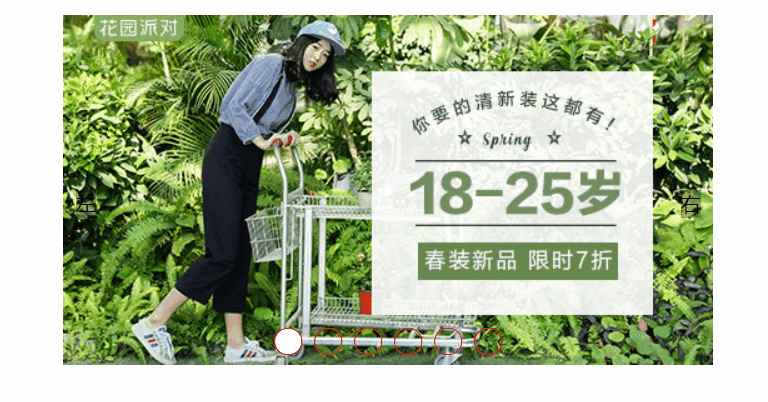
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0px;
padding: 0px;
list-style: none;
}
div {
width: 520px;
height: 280px;
margin: 100px auto;
position: relative;
}
ul li {
width: 520px;
height: 280px;
position: absolute;
top: 0px;
left: 0px;
cursor: pointer;
opacity: 0;
}
ul li.current {
opacity: 1;
}
ol {
width: 182px;
height: 30px;
position: absolute;
left: 50%;
bottom: 0px;
transform: translateX(-91px);
overflow: hidden;
}
ol li {
width: 20px;
height: 20px;
background: rgba(0, 0, 0, 0);
border-radius: 50%;
border: 1px solid red;
float: left;
margin-right: 10px;
cursor: pointer;
}
ol li.current {
background: white;
}
ol li:nth-of-type(6) {
margin-right: 0px;
}
span {
position: absolute;
background: rgba(0, 0, 0, 0.3);
height: 22px;
width: 16px;
top: 50%;
cursor: pointer;
text-align: center;
line-height: 22px;
}
span:nth-of-type(1) {
left: 10px;
}
span:nth-of-type(2) {
right: 10px;
}
</style>
</head>
<body>
<div>
<ul>
<li class="current"><img src="../images/one.jpg" alt=""></li>
<li><img src="../images/two.jpg" alt=""></li>
<li><img src="../images/three.jpg" alt=""></li>
<li><img src="../images/four.jpg" alt=""></li>
<li><img src="../images/five.jpg" alt=""></li>
<li><img src="../images/six.jpg" alt=""></li>
</ul>
<ol>
<li class="current"></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
</ol>
<span>左</span>
<span>右</span>
</div>
<script src="../js/jquery.min.js"></script>
<script>
var num = -1;
var timer;
function move() {
clearInterval(timer);
timer = setInterval(function () {
num++;
if (num > 5) {
num = 0;
}
$('ul li').eq(num).addClass('current');
$('ul li').eq(num).siblings().removeClass('current');
$('ol li').eq(num).addClass('current');
$('ol li').eq(num).siblings().removeClass('current');
}, 500);
}
move();
$('ol li').click(function () {
num = $(this).index();
$(this).addClass('current');
$(this).siblings().removeClass('current');
$('ul li').eq($(this).index()).addClass('current');
$('ul li').eq($(this).index()).siblings().removeClass('current');
});
$('span').eq(0).click(function () {
if (num <= 0) {
// :last 选择器选取最后一个元素。
$('ul li:last').addClass('current');
$('ul li:last').siblings().removeClass('current');
$('ol li:last').addClass('current');
$('ol li:last').siblings().removeClass('current');
num = 5;
}
else {
num--;
$('ul li').eq(num).addClass('current');
$('ul li').eq(num).siblings().removeClass('current');
$('ol li').eq(num).addClass('current');
$('ol li').eq(num).siblings().removeClass('current');
}
});
$('span').eq(1).click(function () {
if (num >= 5) {
// :first 选择器选取第一个元素。
$('ul li:first').addClass('current');
$('ul li:first').siblings().removeClass('current');
$('ol li:first').addClass('current');
$('ol li:first').siblings().removeClass('current');
num = 0;
}
else {
num++;
$('ul li').eq(num).addClass('current');
$('ul li').eq(num).siblings().removeClass('current');
$('ol li').eq(num).addClass('current');
$('ol li').eq(num).siblings().removeClass('current');
}
});
$('div').mouseenter(function(){
clearInterval(timer);
});
$('div').mouseleave(function(){
move();
});
</script>
</body>
</html>
五、微博
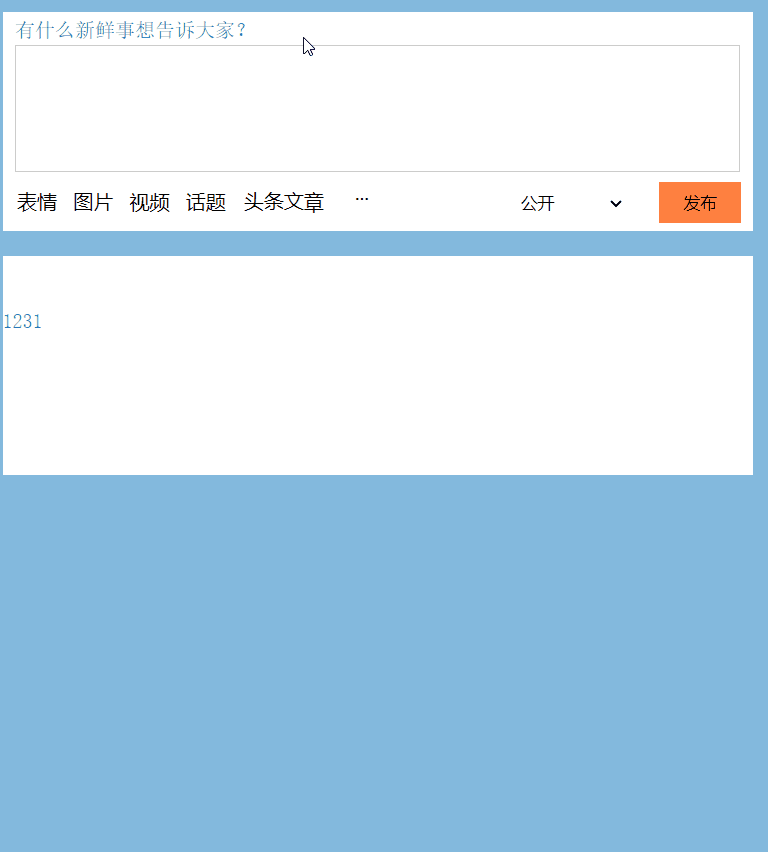
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0px;
padding: 0px;
list-style: none;
}
body {
background: #83b9dd;
}
div {
width: 600px;
height: 175px;
background: white;
margin: 20px auto;
overflow: hidden;
}
span {
color: #1b7fb6;
font-family: "宋体";
line-height: 30px;
display: block;
width: 590px;
height: 26px;
margin-left: 10px;
float: left;
}
textarea {
outline: none;
border-color: #cccccc;
resize: none;
margin-left: 10px;
width: 578px;
height: 100px;
background: rgb(255, 255, 255);
}
div ul {
width: 581px;
float: left;
height: 30px;
margin-left: 10px;
}
div li {
height: 30px;
width: 35px;
float: left;
margin-right: 10px;
text-align: center;
line-height: 39px;
font-size: 16px;
}
div li:nth-of-type(5) {
width: 70px;
}
div li:nth-of-type(6) {
line-height: 24px;
}
div li:nth-of-type(7),
div li:nth-of-type(8) {
float: right;
}
div li:nth-of-type(7) {
width: 66px;
height: 39px;
margin-right: 0px;
line-height: 39px;
}
div li:nth-of-type(8) {
line-height: 39px;
width: 120px;
}
div li:nth-of-type(8) select {
outline: none;
border-style: none;
}
button {
width: 66px;
height: 33px;
background: #ff8140;
border-style: none;
appearance: none;
}
section {
width: 600px;
height: 1500px;
background: #83b9dd;
margin: 20px auto;
}
section ul {
width: 600px;
height: 175px;
margin: 20px auto;
}
section li {
width: 600px;
height: 175px;
background: white;
margin: 20px auto;
overflow: hidden;
cursor: pointer;
}
section li span {
width: 600px;
height: 100px;
margin: 38px auto;
text-align: left;
word-break: break-all;
}
</style>
</head>
<body>
<div>
<span>有什么新鲜事想告诉大家?</span>
<textarea name="" id="txt" cols="30" rows="10"></textarea>
<ul>
<li>表情</li>
<li>图片</li>
<li>视频</li>
<li>话题</li>
<li>头条文章</li>
<li>...</li>
<li><button>发布</button></li>
<li>
<select name="" id="">
<option value="">公开</option>
<option value="">粉丝</option>
<option value="">好友圈</option>
<option value="">仅自己可见</option>
<option value="">群可见</option>
</select>
</li>
</ul>
</div>
<section>
<ul>
<li>
<span>1231</span>
</li>
</ul>
</section>
<script src="../js/jquery.min.js"></script>
<script>
function del(article) {
$(article).remove();
}
$('span').eq(1).parent().click(function () {
del($('span').eq(1).parent());
});
$('button').click(function () {
var txt = $('textarea').val();
if (txt == '') {
alert('内容为空请重新输入');
}
else {
var newList = $(`<li>
<span>${txt}</span>
</li>`);
$(newList).click(function () {
del(this);
});
if ($('ul').eq(1).children().length == 0) {
$('ul').append(newList);
$('textarea').val('')
}
else {
$('ul').eq(1).children().before(newList);
$('textarea').val('')
}
}
});
</script>
</body>
</html>
六、选项卡
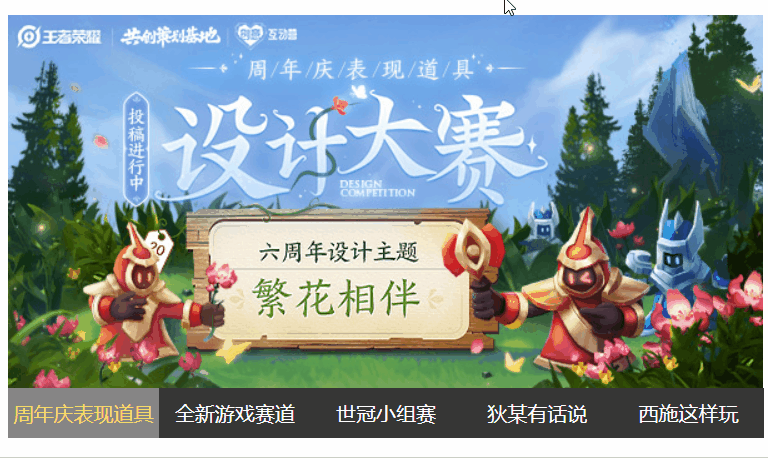
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
padding: 0px;
margin: 0px;
list-style: none;
}
div {
width: 605px;
height: 338px;
margin: 120px auto;
position: relative;
}
ul {
overflow: hidden;
height: 40px;
width: 605px;
position: absolute;
top: 298px;
left: 0px;
}
li {
float: left;
width: 121px;
height: 40px;
background: #363636;
/* color: #F3C258; */
cursor: pointer;
color: white;
line-height: 40px;
text-align: center;
}
li:nth-of-type(1) {
background: rgba(54, 54, 54, 0.6);
color: #ffda69;
}
img {
position: absolute;
top: 0px;
left: 0px;
}
</style>
</head>
<body>
<div>
<ul>
<li>周年庆表现道具</li>
<li>全新游戏赛道</li>
<li>世冠小组赛</li>
<li>狄某有话说</li>
<li>西施这样玩</li>
</ul>
<img src="../images/pic01.png" alt="">
<img src="../images/pic02.png" alt="">
<img src="../images/pic03.png" alt="">
<img src="../images/pic04.png" alt="">
<img src="../images/pic05.png" alt="">
</div>
<script src="../js/jquery.min.js"></script>
<script>
$('img').eq(0).siblings('img').hide();
$('li').click(function () {
$(this).css({ 'background': 'rgba(54,54,54,0.6)', 'color': '#ffda69' });
$(this).siblings().css({ 'background': 'rgb(54,54,54)', 'color': 'white' });
$('img').eq($(this).index()).show();
$('img').eq($(this).index()).siblings('img').hide();
});
</script>
</body>
</html>